Lesson 3 - JSON - Parse, Stringify
JSON - What and Why
What
JSON stands for JavaScript Object Notation. It is a syntax for soring and exchanging data. JSON is essentially text, written in JavaScript object notation. It is a lightweight data interchange format that is self describing and easy to understand. It also has the advantage of being language independant so it can be read and used by ANY programming language.
Why
All data exchanged beteen the browser and server can only be text. JSON can be used to convert JavaScript to text or text in JSON format to JavaScript. This allows data to be worked with as JavaScript objects without complicated parsing or translations.
Syntax
JSON Syntax Rules:
- Data is in name/value pairs
- name and value pairs are seperated by colons "name":"value"
- Data is seperated by commas
- Curly braces hold objects
- Square brackets onld arrays
- Names require double quote "name":"value"
JSON vs JavaScript
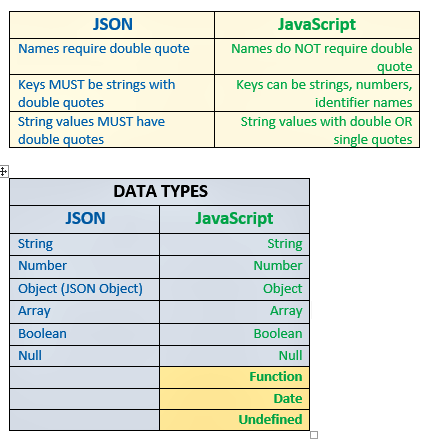
JSON Data Types
- String - double quotes
- {"name":"Mary"}
- Numbers - integer or floating point
- {"apt":36}
- Objects - JSON
- {
- "resident":{"name":"Mary", "apt":36}
- }
- Array - square brackets
- "currentResidents":["Mary", "John", "Sue"]
- Booleans - True/False
- {"occupied":true}
- -->NOTE: There are no quotations around true because it is the value NOT a string
- Null
- {"lateFee": null}
JSON Parse
Data coming from a web server is always in a string format. Use parse to convert string into JavaScript Object.
Syntax
JSON.parse()
Example
- Text from Server
- '{"name":"Mary","apt":36}'
- Code
- var resident = JSON.parse('{"name":"Mary","apt":36}');
- Results in JavaScript Object
- resident.name = "Mary";
- resident.apt = 36;
JSON Stringify
In order to send data to a browser it must be in string format. To convert a JavaScript object into a string we use stringify.
Syntax
JSON.stringify()
Example
- JavaScript Object to String
- var residentString = JSON.stringify(resident);
- residentString = "{"name":"Mary", "apt":36}'
Exceptions Dates and Functions
Dates and functions also must be converted to strings in order to be passed to a server. When dates and functions are passed back from a server we can use a reviver.
Reviver
A reviver is used to transform the value after it is parsed but before it is returned. All of the properties (starting with the most nested) and the value are individually run through the reviver. The reviver will return the value unless the reviver function returns "undefined" the property is deleted from the object. If the receiver transforms only some of the values, be sure to indicate that all other values be returned as-is so they won't be deleted from the object.
Syntax
JSON.parse(text[, reviver])text the string to parse as JSON
reviver this dictates how the value originlly produced by parsing is transformed before being returned.
Examples
-
- var residentString = '{"name":"Mary", "apt":36, "leaseDate":"2012-10-15"}
- var resident = JSON.parse(residentString, function (key,value){
- if (key==="leaseDate"){
- return new Date(value);
- }
- else{
- return value;
- }}),